Note
Go to the end to download the full example code.
Optimize the shape of volumetric mesh¶
graphlow is a powerful tool for shape optimization, leveraging differentiable tensor-based geometric computations.
This example shows how to minimize surface area while constraining volume changes and vertex deformation.
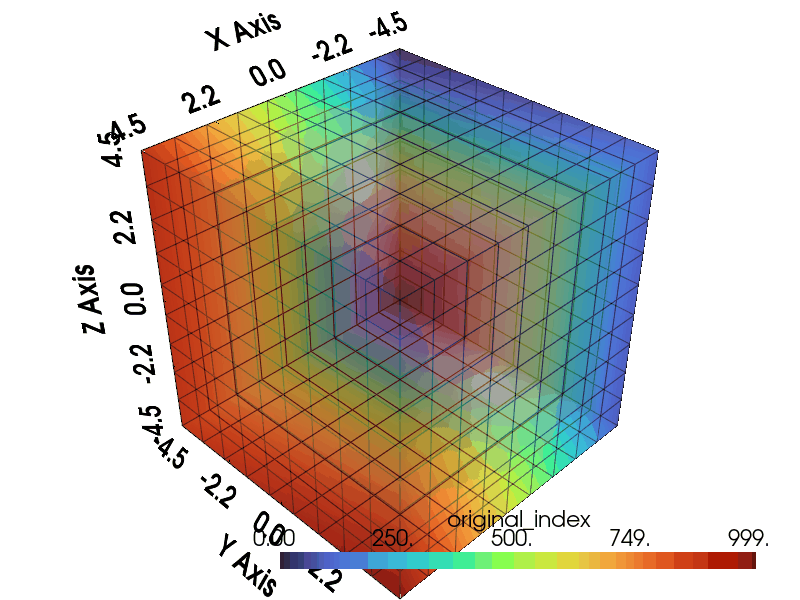
Import necessary modules including graphlow
.
import itertools
import numpy as np
import pyvista as pv
import torch
import graphlow
Prepare a volumetric mesh¶
First, we define a function to generate grid data as the example mesh. If you wish to use your own mesh, you can skip this step.
def generate_grid(ni: int, nj: int, nk: int) -> pv.UnstructuredGrid:
n_cells = (ni - 1) * (nj - 1) * (nk - 1)
x = np.arange(ni, dtype=np.float32)
y = np.arange(nj, dtype=np.float32)
z = np.arange(nk, dtype=np.float32)
X, Y, Z = np.meshgrid(x, y, z, indexing="ij")
points = np.array([X.ravel(), Y.ravel(), Z.ravel()]).T
indices = np.arange(ni * nj * nk).reshape(ni, nj, nk)
hex_lists = []
for k in range(nk - 1):
for j in range(nj - 1):
for i in range(ni - 1):
# hex
v0 = indices[i, j, k]
v1 = indices[i + 1, j, k]
v2 = indices[i + 1, j + 1, k]
v3 = indices[i, j + 1, k]
v4 = indices[i, j, k + 1]
v5 = indices[i + 1, j, k + 1]
v6 = indices[i + 1, j + 1, k + 1]
v7 = indices[i, j + 1, k + 1]
hex_lists.append([8, v0, v1, v2, v3, v4, v5, v6, v7])
hex_cells = list(itertools.chain.from_iterable(hex_lists))
celltypes = np.full((n_cells), pv.CellType.HEXAHEDRON)
return pv.UnstructuredGrid(hex_cells, celltypes, points)
Define the cost function¶
To minimize surface area with constraints, we defined the cost function as follows:
weight_deformation_constraint = 1.0
weight_volume_constraint = 10.0
def cost_function(
mesh: graphlow.GraphlowMesh,
init_total_volume: float,
init_surface_total_area: float,
) -> torch.Tensor:
deformation = mesh.dict_point_tensor["deformation"]
surface = mesh.extract_surface(pass_point_data=True)
volumes = mesh.compute_volumes()
areas = surface.compute_areas()
total_volume = torch.sum(volumes)
total_area = torch.sum(areas)
if torch.any(volumes < 1e-3 * init_total_volume / mesh.n_cells):
return None
cost_area = total_area / init_surface_total_area
volume_constraint = (
(total_volume - init_total_volume) / init_total_volume
) ** 2
deformation_constraint = torch.mean(deformation * deformation)
return (
cost_area
+ weight_volume_constraint * volume_constraint
+ weight_deformation_constraint * deformation_constraint
)
Visualize¶
Following code generate gif plotter to visualize the result.
def create_gif_plotter(mesh: pv.UnstructuredGrid) -> pv.Plotter:
plotter = pv.Plotter(window_size=[800, 600])
init_mesh = mesh.copy()
plotter.add_mesh(init_mesh, show_edges=True, color="white", opacity=0.1)
plotter.add_mesh(
mesh, show_edges=True, lighting=False, cmap="turbo", opacity=0.8
)
plotter.open_gif("shape_optimization_result.gif")
plotter.show_bounds(init_mesh, location="outer")
plotter.camera_position = "iso"
return plotter
Once the cost function and the visualizing function are determined, the remaining step is to write the optimization code that updates the points and deformations
This is the example of the optimization code.
def optimize_shape(input_mesh: pv.UnstructuredGrid):
# Optimization setting
n_optimization = 2000
print_period = int(n_optimization / 100)
n_hidden = 64
deformation_factor = 1.0
lr = 1e-2
output_activation = torch.nn.Identity()
# Initialize
input_mesh.points = input_mesh.points - np.mean(
input_mesh.points, axis=0, keepdims=True
) # Center mesh position
mesh = graphlow.GraphlowMesh(input_mesh)
init_volumes = mesh.compute_volumes().clone()
init_total_volume = torch.sum(init_volumes)
init_points = mesh.points.clone()
init_surface = mesh.extract_surface()
init_surface_areas = init_surface.compute_areas().clone()
init_surface_total_area = torch.sum(init_surface_areas)
w1 = torch.nn.Parameter(torch.randn(3, n_hidden) / n_hidden**0.5)
w2 = torch.nn.Parameter(torch.randn(n_hidden, 3) / n_hidden**0.5)
params = [w1, w2]
optimizer = torch.optim.Adam(params, lr=lr)
def compute_deformation(points: torch.Tensor) -> torch.Tensor:
hidden = torch.tanh(torch.einsum("np,pq->nq", points, w1))
deformation = output_activation(torch.einsum("np,pq->nq", hidden, w2))
return deformation_factor * deformation
deformation = compute_deformation(init_points)
mesh.dict_point_tensor.update({"deformation": deformation}, overwrite=True)
mesh.copy_features_to_pyvista(overwrite=True)
mesh.pvmesh.points = mesh.points.detach().numpy()
plotter = create_gif_plotter(mesh.pvmesh)
plotter.write_frame()
# Optimization loop
print(f"\ninitial volume: {torch.sum(mesh.compute_volumes()):.5f}")
print(" i, cost")
for i in range(1, n_optimization + 1):
optimizer.zero_grad()
deformation = compute_deformation(init_points)
deformed_points = init_points + deformation
mesh.dict_point_tensor.update(
{"deformation": deformation}, overwrite=True
)
mesh.dict_point_tensor.update(
{"points": deformed_points}, overwrite=True
)
cost = cost_function(mesh, init_total_volume, init_surface_total_area)
if cost is None:
deformation_factor = deformation_factor * 0.9
print(f"update deformation_factor: {deformation_factor}")
continue
if i % print_period == 0:
print(f"{i:6d}, {cost:.5e}")
mesh.copy_features_to_pyvista(overwrite=True)
mesh.pvmesh.points = mesh.points.detach().numpy()
plotter.write_frame()
cost.backward()
optimizer.step()
plotter.close()
Run the optimization¶
Finally, we define the main function to run the optimization.
def main():
mesh = generate_grid(10, 10, 10)
optimize_shape(mesh)
if __name__ == "__main__":
main()
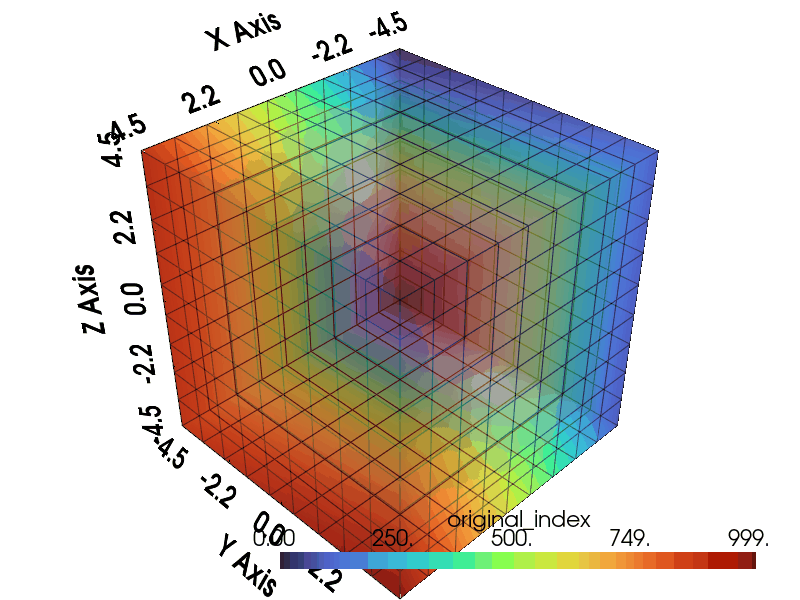
initial volume: 729.00000
i, cost
20, 1.01448e+00
40, 9.67667e-01
60, 9.49057e-01
80, 9.38924e-01
100, 9.31866e-01
120, 9.27344e-01
140, 9.24390e-01
160, 9.22268e-01
180, 9.20598e-01
200, 9.19184e-01
220, 9.17938e-01
240, 9.16834e-01
260, 9.15838e-01
280, 9.14931e-01
300, 9.14106e-01
320, 9.13356e-01
340, 9.12675e-01
360, 9.12268e-01
380, 9.12158e-01
400, 9.11164e-01
420, 9.10699e-01
440, 9.10392e-01
460, 9.12299e-01
480, 9.10039e-01
500, 9.09451e-01
520, 9.09160e-01
540, 9.15024e-01
560, 9.09510e-01
580, 9.08501e-01
600, 9.08273e-01
620, 9.08338e-01
640, 9.13041e-01
660, 9.08037e-01
680, 9.07563e-01
700, 9.07239e-01
720, 9.06996e-01
740, 9.30462e-01
760, 9.08987e-01
780, 9.06644e-01
800, 9.06131e-01
820, 9.05867e-01
840, 9.05617e-01
860, 9.16856e-01
880, 9.06617e-01
900, 9.05196e-01
920, 9.04900e-01
940, 9.04711e-01
960, 9.04511e-01
980, 9.04322e-01
1000, 9.05263e-01
1020, 9.08493e-01
1040, 9.04526e-01
1060, 9.03842e-01
1080, 9.03701e-01
1100, 9.03528e-01
1120, 9.03374e-01
1140, 9.03223e-01
1160, 9.03076e-01
1180, 9.02960e-01
1200, 9.11570e-01
1220, 9.04671e-01
1240, 9.03029e-01
1260, 9.02668e-01
1280, 9.02540e-01
1300, 9.02424e-01
1320, 9.02316e-01
1340, 9.02211e-01
1360, 9.02108e-01
1380, 9.02009e-01
1400, 9.15329e-01
1420, 9.05777e-01
1440, 9.02112e-01
1460, 9.01904e-01
1480, 9.01790e-01
1500, 9.01699e-01
1520, 9.01613e-01
1540, 9.01529e-01
1560, 9.01446e-01
1580, 9.01363e-01
1600, 9.01280e-01
1620, 9.01546e-01
1640, 9.06637e-01
1660, 9.01269e-01
1680, 9.01200e-01
1700, 9.01106e-01
1720, 9.01028e-01
1740, 9.00944e-01
1760, 9.00862e-01
1780, 9.00780e-01
1800, 9.00696e-01
1820, 9.00611e-01
1840, 9.00525e-01
1860, 9.00510e-01
1880, 9.10021e-01
1900, 9.02247e-01
1920, 9.00738e-01
1940, 9.00346e-01
1960, 9.00226e-01
1980, 9.00133e-01
2000, 9.00040e-01
Total running time of the script: (0 minutes 42.095 seconds)